Orthographic Now in Development
This week marks the return of the development of Orthographic. Orthographic was originally going to be made with RPG Maker VX Ace, but I was starting to code more in Java while I was writing the script, so I quickly lost interest in development. When they released RPG Maker MV with the JavaScript plugin system, I decided to make Orthographic my Senior Project. I like JavaScript, and let's face it, Ruby sucks. I am going to write the script, get all the voice, write all the plugins I use myself, and design the entire game. Hopefully I will get most of it done during the Summer. This week I only worked on it for about an hour, and I started two plugins. **Don't want to read this whole thing but still want to know how these plugins work? I streamed the development of the whole process, so you can just watch that!
TCG Custom Title Screen
In pretty much every game I'be ever made, I put my name on the title screen. RPG Maker does not allow something like this without plugins. Only using my own plugins, I wrote one myself. The first step in making an RPG Maker MV plugin is to add the comments in the top. The top comments declare the name of the plugin, the parameters, and the help file. They go at the top of the file like this:
/*: * * @plugindesc Allows for custom title screen * * @author José Rodriguez-Rivas * * @param Credit at Bottom * @desc Set to "true" to draw the credit at the bottom of the title screen * @default true * * * @param Prefix Credit * @desc Text drawn before name in credit. * @default Created By * * * @param Developer Name * @desc Will write "[Prefix] [name]" at bottom of Title Screen * @default Foo Bar * * @help * Allows for the user to able to customize the title screen of their game. * */
As you can see, my custom menu has three parameters so far. All these parameters are for the credit at the bottom. The first parameter is a boolean that determines whether or not to even draw the credit at the bottom. The next parameter is a string that tells what text is drawn before the name of the creator. The default is "Created by" but I wanted to make sure to include customization in the plugin, so if someone wanted to say "Developer:" or "Developed by" instead, they can. The third parameter is the name of the developer. Pretty straight forward, they enter their name.
The JavaScript itself is wrapped in
(function() { // Code here })();
So that I can make any variable I want, that will only exist in the scope of the plugin. The first thing I do in my plugin is declare all the variables I am going to need. The first variable is meant to store the pre-written function I am modifying. This is always a good idea because it makes sure that any other plugin that wants to use that same function can still work. That code looks like this:
var _Scene_Title_createForeground = Scene_Title.prototype.createForeground;
Scene_Title is the built in class that handles drawing the title screen of any RPG Maker MV game. Next I extract the given parameters into variables. All plugin parameters are kept in a static array that reads from a js file generated by the editor itself. The array contains an object that is identified by the file name of the plugin, excluding the .js at the end. Getting the parameters looks like this;
var parameters = PluginManager.parameters("TCGCustomTitleScreen"); var devName = String(parameters["Developer Name"]); var credPre = String(parameters["Prefix Credit"]); var drawCredit = String(parameters["Credit at Bottom"]) == "true";
Next is rewriting the prototype of the function I need in order for my script to work, in this case, it is the function that created the foreground of the title screen.
Scene_Title.prototype.createForeground = function() { //Code goes here };
Now that I have that, I can start modifying what the function does, adding in my own functionality. First thing to do is call the stored function, so that everything that was supposed to happen still does
_Scene_Title_createForeground.call(this);
Next thing to do is add my own code, in this case, I need to create a Sprite object, that will handle drawing the text, and if the user set the drawCredit parameter to true, draw the text.
this._devNameSprite = new Sprite(new Bitmap(Graphics.width, Graphics.height)); this.addChild(this._devNameSprite); if(drawCredit) { this.drawDeveloperName(); }
"drawDeveloperName" is a function I declare later in the plugin. So I initialized the sprite to be the size of the screen, add it to the screen, and if the user wants, draw the text. I should probably move all of that into that if statement, to save some memory when the user does not want the text. Lastly, I just need the code that draws the text. Using the parameters given, the "drawDeveloperName" function draws the credit at the bottom left of the screen like this:
Scene_Title.prototype.drawDeveloperName = function() { var x = 6; var y = Graphics.height - 28; var maxWidth = Graphics.width - x * 2; var text = credPre + " " + devName; this._devNameSprite.bitmap.fontSize = 30; this._devNameSprite.bitmap.drawText(text, x, y, maxWidth, 12, 'left'); };
Pretty simple right? I will, of course, add more stuff to customize to the plugin later on, like changing how the title of the game is drawn. The full plugin so far looks like this:
/*: * * @plugindesc Allows for custom title screen * * @author José Rodriguez-Rivas * * @param Credit at Bottom * @desc Set to "true" to draw the credit at the bottom of the title screen * @default true * * * @param Prefix Credit * @desc Text drawn before name in credit. * @default Created By * * * @param Developer Name * @desc Will write "[Prefix] [name]" at bottom of Title Screen * @default Foo Bar * * @help * Allows for the user to able to customize the title screen of their game. * */ (function() { var _Scene_Title_createForeground = Scene_Title.prototype.createForeground; var parameters = PluginManager.parameters("TCGCustomTitleScreen"); var devName = String(parameters["Developer Name"]); var credPre = String(parameters["Prefix Credit"]); var drawCredit = String(parameters["Credit at Bottom"]) == "true"; Scene_Title.prototype.createForeground = function() { _Scene_Title_createForeground.call(this); this._devNameSprite = new Sprite(new Bitmap(Graphics.width, Graphics.height)); this.addChild(this._devNameSprite); if(drawCredit) { this.drawDeveloperName(); } }; Scene_Title.prototype.drawDeveloperName = function() { var x = 6; var y = Graphics.height - 28; var maxWidth = Graphics.width - x * 2; var text = credPre + " " + devName; this._devNameSprite.bitmap.fontSize = 30; this._devNameSprite.bitmap.drawText(text, x, y, maxWidth, 12, 'left'); }; })();
TCG Dungeons
Orthographic is going to be dungeon based, sort of like Zelda style. For that, I need some special code to run only in dungeons. I do this through note tags. So far, I only have the script writing the name of the dungeon at the top left corner of the screen. My comments at the top can use some work, but here they are
/*: * * @plugindesc Displays Dungeon information in a dungeon * * @author José Rodriguez-Rivas * * @help * Displays Dungeon information in a dungeon * */
Perhaps in the comments someone can help me be more descriptive? Anyways for this plugin, I need the Scene_Map class, which handles drawing the world. The two functions I need are the start function, to let me initialize and add my sprite, and the update function, that lets me draw the text. First things first, I need to store the functions in their own variables to call when I start rewriting the function
var _Scene_Map_start = Scene_Map.prototype.start; var _Scene_Map_update = Scene_Map.prototype.update;
Next, I need to create the start function, I am going to put the entire function, because I already explained most of what's going on above
Scene_Map.prototype.start = function() { _Scene_Map_start.call(this); this._dSprite = new Sprite(new Bitmap(Graphics.width, Graphics.height)); this.addChild(this._dSprite); };
I just call the existing function, and add a sprite. I do not allow the ability to turn the drawing on or off, because the user can simply turn off the plugin if they don't want it. Anyways, next I rewrite the update function. Before you read this, know that putting an empty or null string in a boolean will act as a false boolean.
Scene_Map.prototype.update = function() { _Scene_Map_update.call(this); if($dataMap.meta.dTitle) { this._dSprite.bitmap.drawText($dataMap.meta.dTitle, 10, 10, Graphics.width, 30, "left"); } };
What is happening is I first call the existing function. the "$dataMap.meta.dTitle" is a note tag from within the editor itself. Adding a note tag to a map will save it in a generated JSON file that the js can access. The structure of a note tag is <title:string>. In my case, the title is "dTitle", and the string is the name of the dungeon. If there is no dTitle note tag, then the plugin assumes that the current map is not a dungeon, and does not draw the text. However, if the note tag is present, it simply draws the title at the top left of the screen. I plan on adding a whole ton of stuff to this plugin, like a whole key system that links to in editor variables and stuff like that, I will keep updates coming. The full plugin so far looks like this;
/*: * * @plugindesc Displays Dungeon information in a dungeon * * @author José Rodriguez-Rivas * * @help * Displays Dungeon information in a dungeon * */ (function() { var _Scene_Map_start = Scene_Map.prototype.start; var _Scene_Map_update = Scene_Map.prototype.update; Scene_Map.prototype.start = function() { _Scene_Map_start.call(this); this._dSprite = new Sprite(new Bitmap(Graphics.width, Graphics.height)); this.addChild(this._dSprite); }; Scene_Map.prototype.update = function() { _Scene_Map_update.call(this); if($dataMap.meta.dTitle) { this._dSprite.bitmap.drawText($dataMap.meta.dTitle, 10, 10, Graphics.width, 30, "left"); } }; })();
So that is what I have gotten done on my senior project so far, it isn't much, but that is only because I just started. I will be streaming all my Twitch, go check me out at https://www.twitch.tv/theanimenerd2009 The past streams will also be posted on YouTube. Feel free to check out the stream where I made all this below!
-José Rodriguez-Rivas
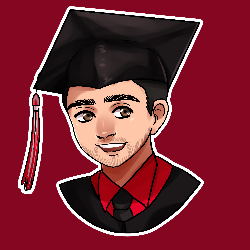