Search Function
I know I posted some lengthy posts on Wednesday, but I really want to keep a regular schedule with writing these posts. Every Friday I will write a post, sometimes during the week, if something comes up, I will write then as well. So always at least once a week.
Anyways, I decided to add a search function to the blog. I have never really had a website that was completely data driven like this one. One advantage to having a data driven site is the ability to search through all the pages. Adding a search function was a pretty simple task.
First things first, I built the form.
<form action="/search"> <div class="input-group"> <span class="input-group-addon" id="search-addon"><i class="fa fa-search"></i></span> <input type="text" class="form-control" placeholder="Search for" id="searchText" name="searchText" aria-describedby="search-addon"> <span class="input-group-btn"> <input type="submit" class="btn btn-default" value="Search"> </span> </div>
</form>
Pretty simple, stylized with Bootstrap, of course. Next was creating the search page. The search page follows the sample template of the other pages on the site. Before doing anything, the search page first checks if there is anything to even search for from the GET request. If there is, store the text to search for in a variable.
if(!isset($_GET["searchText"]) || empty($_GET["searchText"])) { header("Location: http://" . $_SERVER["HTTP_HOST"] . "/"); } else { $searchText = $_GET["searchText"]; }
This makes sure that someone doesn't just enter nothing into the search bar, or tries to access the search page without even using the form, which would break the page. Next is to make sure that the search string is "SQL friendly" meaning escaping any characters that could break the query. Luckily, mysqli already has a method that does this, all you need to do is pass in a connection, and a string.
$searchTextSql = mysqli_real_escape_string($conn, $searchText);
That method essentially takes any characters like ' and " and converts them to escape characters so that the string can be used in a query. Now, to generate the sql and get a result.
$dateFormat = "DATE_FORMAT(publishDate, '%W, %M %D')"; //I like to put date formats in their own variables to make accessing them easier. $searchSql = "SELECT id, postTitle, LEFT(postText, 75), $dateFormat, category FROM posts WHERE publishDate <= CURRENT_DATE() AND (postTitle LIKE '%$searchTextSql%' OR postText LIKE '%$searchTextSql%') ORDER BY dateCreated desc"; $searchResult = mysqli_query($conn, $searchSql);
So basically what this query does is find the information of a post where the publish date is less than or eqal to today, meaning it is published, and to use the "LIKE" keyword to see what posts have the given text in their titles or content. The next bit of code, that I will not show, is to use that information to build links. If the result set has no rows, it echos a link with a blank href that says that nothing was found. If the result set has one or more rows, the php generates links that link to the proper blog page for the category. The links look like the same links on the index of each category, but with the category icon before the title.
Searching makes blogs and most other kinds of websites much easier to use by the user. They can quickly find whatever they are looking for in the site without having to navigate through so many pages. If you are going to build a data driven site, it is crucial that you add in a search function.
-José Rodriguez-Rivas
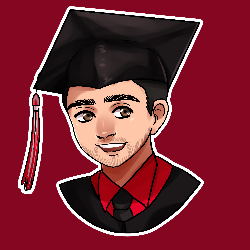