Small Change in Plugin
I added a very basic functionality to the Dungeons plugin. I want my dungeons to have a key system, similar to Zelda. Doing that is pretty easy, I just have a variable for each dungeon with the amount of keys that the player currently has. Then, the plugin draws the amount of keys under the name of the dungeon. The maps have to point the plugin to the variable in a note tag called "keys". The first problem I faced was the bitmap not clearing. Say, for example, a player has 3 keys when they walk into a dungeon, and they use one. Without clearing the bitmap, the text saying there's 2 keys left would be drawn on top of the text saying there's 2 keys left. So before drawing on update, I clear the bitmap with
this._dSprite.bitmap.clear();
After checking if the notetag exists, I cast it to a number.
var keyNum = Number($dataMap.meta.keys);
Next, I use the id to get the amount of keys to draw onto the screen. The game variables are in a $gameVariables object, and a variables value is retrieved from the value(id) method in the object. I get the number of keys from the id in the notetag like this
var keys = $gameVariables.value(keyNum);
Then I draw the amount of keys to the screen
this._dSprite.bitmap.drawText("Keys: " + keys, 10, 40, Graphics.width, 30, "left");
All the new code put together looks like this
this._dSprite.bitmap.clear(); // Draw Dungeon Name Code if($dataMap.meta.keys) { var keyNum = Number($dataMap.meta.keys); var keys = $gameVariables.value(keyNum); this._dSprite.bitmap.drawText("Keys: " + keys, 10, 40, Graphics.width, 30, "left"); }
I also started writing the script, the stream can be found below.
-José Rodriguez-Rivas
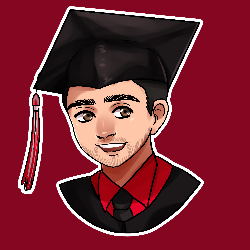